osCommerce integration
A free online shop program featuring order history, shopping carts, full search capability, product reviews, secure transactions, bestseller lists, and related items.
Integration with osCommerce is made by placing sale tracking script into the confirmation page. To obtain the values of OrderID and TotalSale, snippet connects to osCommerce database and retrieves the values from there.
How to integrate PostAffiliatePro with osCommerce
Find file checkout_success.php
Find and open file checkout_success.php in osCommerce source files.
Locate right place for integration
Inside the file find this line:
if ($global['global\_product\_notifications'] != '1') {...
it should be somewhere after this line:
<! DOCTYPE ........>
Add integration code
Insert the following code just above that line:
//--------------------------------------------------------------------------
// integration code
//--------------------------------------------------------------------------
// get order id
$sql = "select orders_id from ".TABLE_ORDERS.
" where customers_id='".(int)$customer_id.
"' order by date_purchased desc limit 1";
$pap_orders_query = tep_db_query($sql);
$pap_orders = tep_db_fetch_array($pap_orders_query);
$pap_order_id = $pap_orders['orders_id'];
// get total amount of order
$sql = "select value from ".TABLE_ORDERS_TOTAL.
" where orders_id='".(int)$pap_order_id.
"' and class='ot_subtotal'";
$pap_orders_total_query = tep_db_query($sql);
$pap_orders_total = tep_db_fetch_array($pap_orders_total_query);
$pap_total_value = $pap_orders_total['value'];
//get product ids
$sql = "select products_id from " .TABLE_ORDERS_PRODUCTS.
" where orders_id=".(int)$pap_order_id;
$pap_orders_products_query = tep_db_query($sql);
$pap_orders_products = '';
while ($row = tep_db_fetch_array($pap_orders_products_query)) {
$pap_orders_products .= $row['products_id'] . ',';
}
$pap_orders_products = substr($pap_orders_products, 0, -1);
// draw invisible image to register sale
if($pap_total_value != "" && $pap_order_id != "")
{
print '<script id="pap_x2s6df8d" src="https://URL_TO_PostAffiliatePro/scripts/trackjs.js" type="text/javascript"></script>
'."<script type=\"text/javascript\">PostAffTracker.setAccountId('Account_ID');
var sale = PostAffTracker.createSale();
sale.setTotalCost('$pap_total_value');
sale.setOrderID('$pap_order_id');
sale.setProductID('$pap_orders_products');
PostAffTracker.register();
</script>";
}
//--------------------------------------------------------------------------
// END of integration code
//--------------------------------------------------------------------------
Integration is finished
It is now integrated. Every time customer enters the order confirmation page the tracking code is called and it will register a sale for referring affiliate.
Another integration
If you plan to divide products into more campaigns, you will probably need another integration, that will divide the whole sale into single product sales.
//--------------------------------------------------------------------------
// integration code
//--------------------------------------------------------------------------
// get order id
$sql = "select orders_id from ".TABLE_ORDERS.
" where customers_id='".(int)$customer_id.
"' order by date_purchased desc limit 1";
$pap_orders_query = tep_db_query($sql);
$pap_orders = tep_db_fetch_array($pap_orders_query);
$pap_order_id = $pap_orders['orders_id'];
//get variables for script
$sql = "select products_id,products_price,products_quantity from " .TABLE_ORDERS_PRODUCTS.
" where orders_id=".(int)$pap_order_id;
$pap_products_total_query = tep_db_query($sql);
$k = 0;
while ($row = tep_db_fetch_array($pap_products_total_query)) {
$pap_products_total[$k+1] = $row['products_price'] * $row['products_quantity'];
$pap_products[$k+1] = $row['products_id'];
$k++;
}
// draw invisible image to register sale
if($pap_order_id != "")
{
?>
<script id="pap_x2s6df8d" src="https://URL_TO_PostAffiliatePro/scripts/trackjs.js" type="text/javascript"></script>
<script type="text/javascript">
PostAffTracker.setAccountId('Account_ID'); <?php
for ($j=1; $j<=$k; $j++){
echo "var sale".$j." = PostAffTracker.createSale();\n".
"sale".$j.".setTotalCost('". $pap_products_total[$j]."');\n".
"sale".$j.".setOrderID('".$pap_order_id."');\n".
"sale".$j.".setProductID('".$pap_products[$j]."');\n\n";
}
?>
PostAffTracker.register();
</script>;
<?php
}
//--------------------------------------------------------------------------
// END of integration code
//--------------------------------------------------------------------------
Direct PayPal integration with osCommerce
If you want to rely on PayPal IPN (as this is secure and 100% sure that the transaction will be recorded), you can directly edit the PayPal button template in osCommerce. Login to your FTP and navigate to catalog/includes/modules/payment/ and edit file paypal_standard.php.
Find function process_button and scroll down to end of it. You should find this block of code:
} else {
reset($parameters);
while (list($key, $value) = each($parameters)) {
$process_button_string .= tep_draw_hidden_field($key, $value);
}
}
return $process_button_string;
// --------------------------------------------
// change this whole block of code to this block:
} else {
reset($parameters);
while (list($key, $value) = each($parameters)) {
if ($key == "custom") {
$tofix = tep_draw_hidden_field($key, $value);
$process_button_string .= substr($tofix,0,-1) .' id="pap_ab78y5t4a" >';
}
else {
$process_button_string .= tep_draw_hidden_field($key, $value);
}
}
}
$process_button_string .= '<script type="text/javascript">';
$process_button_string .= 'document.write(unescape("%3Cscript id=%27pap_x2s6df8d%27 src=%27" + (("https:" == document.location.protocol) ? "https://" : "http://") + "URL_TO_PostAffiliatePro/scripts/trackjs.js%27 type=%27text/javascript%27%3E%3C/script%3E"));';
$process_button_string .= '</script><script type="text/javascript">PostAffTracker.setAccountId(\'default1\');';
$process_button_string .= 'PostAffTracker.setAppendValuesToField(\'||\');';
$process_button_string .= 'PostAffTracker.writeCookieToCustomField(\'pap_ab78y5t4a\');</script>';
return $process_button_string;
This will add tracking script directly to the paypal button and insert proper value into custom parameter.
Now, you have to resend the IPN from osCommerce to PAP too. See the next step.
PayPal redirect to PAP
When there is a sale, PayPal sends IPN to your osCommerce. You have to resend it to PAP to save the transaction. Navigate to catalog/ext/modules/payment/paypal/ in your FTP and modify file standard_ipn.php . Insert the following code to the beginning of the file:
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, "https://URL_TO_PostAffiliatePro/plugins/PayPal/paypal.php");
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS, $_POST);
curl_exec($ch);
The last step is to modify customer ID saved in custom field, to the the value without PAP visitor ID. Find line:
if ($result == 'VERIFIED') {
and add the following code above the line:
$separator = '||';
if ($_POST['custom'] != '') {
$explodedCustomValue = explode($separator, $_POST['custom'], 2);
if (count($explodedCustomValue) == 2) {
$_REQUEST['custom'] = $_POST['custom'] = $explodedCustomValue[0];
$HTTP_POST_VARS['custom'] = $explodedCustomValue[0];
}
}
Do not forget to integrate your website with the click tracking code.
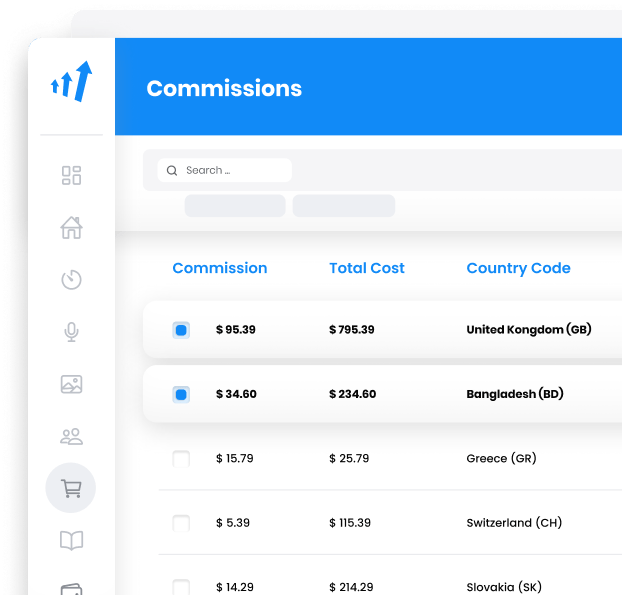
What is osCommerce?
osCommerce is a free, open-source eCommerce platform and shopping cart solution designed to help businesses manage and sell products online efficiently. Founded in March 2000 as The Exchange Project, it is built on PHP and MySQL technologies. osCommerce offers a range of features, including zero platform processing fees, search engine optimization (SEO), mobile-friendly design, GDPR compliance, and seamless integration with payment gateways like PayPal and Stripe. It caters to both B2C and B2B clients, making it a versatile choice for various types of online retailers.
osCommerce began as an informal project by Harald Ponce de Leon and evolved into a widely used eCommerce solution, peaking in popularity around 2009. Despite its early success, the platform saw a decline due to a lack of commercial strategy, leading to near obsolescence by 2020. In 2021, the Holbi Group acquired osCommerce and released osCommerce 4, introducing significant updates and modernizing the platform to meet current market demands.
osCommerce targets small to medium-sized businesses, especially those with technical expertise or access to affordable development support. It is ideal for entrepreneurs who prefer open-source software to minimize initial investments and avoid ongoing costs. The platform’s flexibility allows businesses to create highly customizable online stores, tailoring the shopping experience to their specific needs without the burden of expensive licensing fees.
Main Features of osCommerce
- Advanced Product Catalog Management: Easily organize, manage, and display products.
- Multilingual and Multi-Currency Support: Reach a global audience by offering multiple languages and currencies.
- Integration with Marketplaces: Connect with popular marketplaces like Amazon and eBay to expand sales channels.
- Extensive Library of Add-Ons: Customize and enhance your store with a wide range of plugins and extensions.
- Open-Source Flexibility: Access and modify the source code for personalized development.
- Cost-Effective Solution: Free to use if self-hosted, with optional costs for hosting services and premium add-ons.
Pricing Options for osCommerce
While osCommerce itself is free to download and use, there are associated costs to consider. Expenses may include domain registration, web hosting, and any additional paid extensions or professional support services. Hosting plans suitable for osCommerce typically range from $4.99/month to $19.99/month, depending on the hosting provider and the required resources.
User Insights on osCommerce
- Pros:
- Fast and efficient performance due to lightweight design.
- High degree of customization thanks to its open-source nature.
- Low hosting requirements, making it a cost-effective option.
- Cons:
- Requires substantial programming knowledge to fully utilize and customize.
- The user interface and some features may feel outdated compared to newer platforms.
Overall, osCommerce is best suited for technically proficient users or businesses with access to development resources. Its flexibility and cost-effectiveness make it an attractive option for those willing to invest time and effort into customizing their online store. However, beginners or those without technical skills might find the platform challenging due to its complexity and the hands-on approach required.
osCommerce Reviews on YouTube
For those interested in visual demonstrations and reviews, here are some helpful YouTube videos:
Alternatives to osCommerce
If you’re considering other options besides osCommerce, here are some popular alternatives:
- WooCommerce – woocommerce.com
- Magento – magento.com
- PrestaShop – prestashop.com
- OpenCart – opencart.com
- Drupal Commerce – drupalcommerce.org
- Joomla! with VirtueMart – virtuemart.net
- Zen Cart – zencart.com
- Sylius – sylius.com
- Spree Commerce – spreecommerce.org
- Shopify – shopify.com
Discover seamless integration with osCSS on Post Affiliate Pro. Our open-source e-commerce solution offers powerful shopping cart functionality under the GNU General Public License. Easily integrate by placing a sale tracking script on the confirmation page to retrieve OrderID and TotalSale values. Join now for a free trial and optimize your affiliate management effortlessly!
Explore seamless integrations with Post Affiliate Pro to enhance your affiliate marketing strategies. Discover solutions for e-commerce, email marketing, payments, and more, with easy integrations for platforms like 1&1 E-Shop, 2Checkout, Abicart, and many others. Optimize your affiliate network with these powerful tools.