Summer Cart integration
An easy-to-use full-featured PHP shopping cart.
Summer Cart has all the tools and features to enable online merchants easily build a working store from top to bottom. Whether you sell a dozen of niche specialties, or list hundreds of mass products, you will get high visibility and popularity of your operation through a masterly crafted store.
How to integrate PostAffiliatePro with Summer Cart
Integration of Post Affiliate Pro with Summer Cart will require to modify not just footer of your shop (to add click tracking code), but also 2 shopping cart classes. See details of this integration below.
Click integration
Click tracking code should be stored into file skins//customer/footer.tmpl.
If you don’t have this file in your skin, just copy it from skeleton directory to your theme directory and add click tracking code, which is prepared for you in Post Affiliate Pro (menu Tools -> Integration -> Click tracking)
Add this code before </body> tag in your footer.tmpl template.
Sale Integration
Sale integration will work in 2 steps and integration is done by calling PAP API requests to Post Affiliate Pro directly from php code of your shopping cart.
In first step will be created transaction in Post Affiliate Pro (status Pending) and later, when order will be delivered to your customer, transaction in Post Affiliate Pro will change status to Approved.
Add transaction step
Your shopping cart will create order in time, when is visitor redirected to payment processor (e.g. Paypal). In same time is created transaction in Post Affiliate Pro (status Pending)
Open the shopping cart file: /include/sc/util/order/OrdersInProgress.php
so that we can place there the sale tracking code right at the end of class scOrdersInProgress .
In order to track the entire order as 1 transaction (commission) in Post Affiliate Pro (even if multiple items are purchased during the particular order) use the following code:
private function registerNewPostAffiliateProTransaction($order) {
include_once('<PATH_TO_PAP_API>/PapApi.class.php');
$saleTracker = new Pap_Api_SaleTracker('https://URL_TO_PostAffiliatePro/scripts/sale.php');
$productIDs = '';
$items = $order->getOrderItems();
foreach($items as $item) {
$productIDs .= $item->get('OrderItemProductCode').',';
}
$sale = $saleTracker->createSale();
$papOrderDetails = $order->getOrderTotalLines();
$sale->setTotalCost($papOrderDetails[0]->get('OrderTotalLineCustomerCurrencyAmount'));
$sale->setOrderID($order->getPK());
$sale->setProductID($productIDs);
$saleTracker->register();
}
If you wish each item purchased during the order to be tracked as a separate transaction (commission) in Post Affiliate Pro, then use the following code:
private function registerNewPostAffiliateProTransaction($order) {
include_once('<PATH_TO_PAP_API>/PapApi.class.php');
$saleTracker = new Pap_Api_SaleTracker('https://URL_TO_PostAffiliatePro/scripts/sale.php');
$items = $order->getOrderItems();
foreach($items as $item) {
$sale = $saleTracker->createSale();
$sale->setTotalCost($item->get('OrderItemTotal'));
$sale->setOrderID($order->getPK());
$sale->setProductID($item->get('OrderItemProductCode'));
}
$saleTracker->register();
}
IMPORTANT: this method should be stored before end of class, it means before last } in file /include/sc/util/order/OrdersInProgress.php
IMPORTANT: Don’t forget to replace with correct path to PapApi.class.php file.
PapApi.class.php file can be downloaded from your Post Affiliate Pro installation in menu Tools-> Integration -> Api Integration.
Copy it to your server, where is installed your shop and set the correct path on place of .
Add transaction step
Now we should use the method registerNewPostAffiliateProTransaction, which we added in previous step.
Please add following line of code into method createOrder right before last line
return $this->_lastOrderId;
$this->registerNewPostAffiliateProTransaction($order);
Change status of transaction
Status of existing transactions in Post Affiliate Pro can be controlled by changing of status in your shopping cart.
Following code will change status of PAP transaction to Approved, if you will set your shopping cart order to status Delivered.
And it will set PAP transaction to status Declined, if you will set your shopping cart order to status Cancelled, Failed Or Returned.
Edit your shopping cart file /include/sc/domainobj/Order.php and at the end of class Order add following method:
private function updatePostAffiliateProTransaction() {
try {
include_once('<PATH_TO_PAP_API>/PapApi.class.php');
$session = new Gpf_Api_Session("https://URL_TO_PostAffiliatePro/scripts/server.php");
if(!$session->login("<MERCHANT_USERNAME>","<MERCHANT_PASSWORD>")) {
return false;
}
$request = new Pap_Api_TransactionsGrid($session);
$request->addFilter("orderid", Gpf_Data_Filter::LIKE, $this->getPK());
$request->addFilter("rtype", Gpf_Data_Filter::EQUALS, 'S');
try {
$request->sendNow();
$grid = $request->getGrid();
$recordset = $grid->getRecordset();
} catch (Exception $e) {
return false;
}
foreach($recordset as $rec) {
$transaction = new Pap_Api_Transaction($session);
$transaction->setTransid($rec->get('transid'));
try {
if(!$transaction->load()) {
return false;
} else {
if ($transaction->getStatus() != 'D') {
$newStatus = '';
switch($this->get('OrderStatus')) {
case scOrderStatus::ORDER_STATUS_UNFINISHED:
case scOrderStatus::ORDER_STATUS_PAYMENT_PENDING:
case scOrderStatus::ORDER_STATUS_NEW:
case scOrderStatus::ORDER_STATUS_IN_PROGRESS:
case scOrderStatus::ORDER_STATUS_ON_HOLD:
case scOrderStatus::ORDER_STATUS_QUEUED:
$newStatus = 'P';
break;
case scOrderStatus::ORDER_STATUS_RETURNED:
case scOrderStatus::ORDER_STATUS_PAYMENT_FAILED:
case scOrderStatus::ORDER_STATUS_CANCELLED:
$newStatus = 'D';
break;
case scOrderStatus::ORDER_STATUS_DELIVERED:
$newStatus = 'A';
break;
default:
return false;
}
// changing the status of a transaction
if (strlen($newStatus) && $transaction->getStatus() != $newStatus) {
$transaction->setStatus($newStatus);
$transaction->save();
}
}
}
} catch (Exception $e) {
return false;
}
}
} catch (Exception $e) {
return false;
}
return true;
}
IMPORTANT: this method should be stored before end of class, it means before last } in file /include/sc/domainobj/Order.php
IMPORTANT: Don’t forget to replace with correct path to PapApi.class.php file.
PapApi.class.php file can be downloaded from your Post Affiliate Pro installation in menu Tools-> Integration -> Api Integration.
Copy it to your server, where is installed your shop and set the correct path on place of .
IMPORTANT: On place of use your merchant username and on place of use your password. API request will use your user credentials to access transactions in your Post Affiliate Pro installation.
Change status of transaction
Now we should use the method, which we added to class Order.
Add the following line of code (found in the box below) into file /include/sc/domainobj/Order.php to the very end of the updateOrderStatus and setOrderStatus methods.
$this->updatePostAffiliateProTransaction();
Custom Order Statuses
In case you plan to use custom order statuses in your Summer Cart, you should adapt method updatePostAffiliateProTransaction in your shopping cart file /include/sc/domainobj/Order.php.
in switch function you should add new case statements, where value will be ID of your custom status.
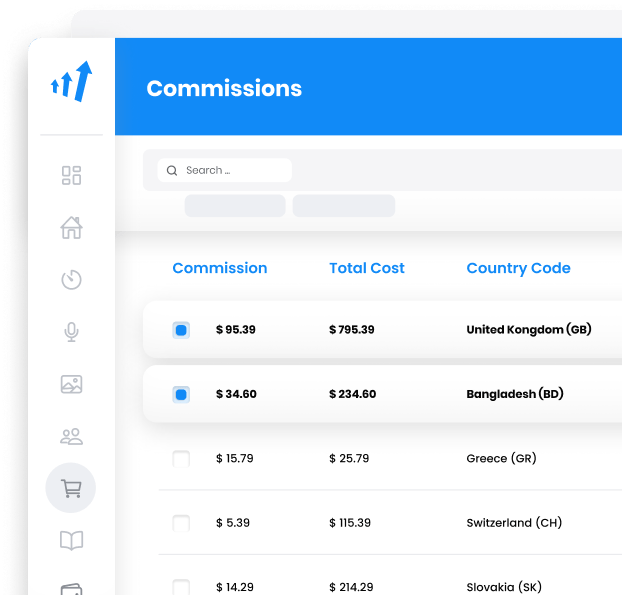
What is Summer Cart?
Summer Cart is a comprehensive e-commerce software solution designed to streamline the creation and management of online stores. It empowers businesses of all sizes to establish a robust online presence with ease. Offering a wide array of features such as product catalog management, order processing, customer relationship management, and integrated marketing tools, Summer Cart serves as an all-in-one platform for aspiring and established e-commerce ventures.
Developed by Mirchev Ideas, a Bulgarian software company founded by Georgi Mirchev, Summer Cart made its debut in 2008. Conceived with the goal of making online retail accessible and cost-effective for businesses in Bulgaria, it quickly gained traction. By 2014, Summer Cart had become the backbone for over 2,700 online stores, demonstrating its versatility and the value it brought to the e-commerce landscape.
Summer Cart primarily caters to small and medium-sized enterprises (SMEs) across diverse industries, including fashion, electronics, and home goods. Its user-friendly interface and extensive feature set make it an ideal choice for businesses seeking an integrated solution. With its mobile-responsive designs, multi-language and multi-currency support, and advanced B2B functionalities, Summer Cart enables businesses to expand their reach and enhance online sales effortlessly.
Key Features of Summer Cart
- SEO Optimization Tools: Enhance your store’s visibility on search engines to attract more customers.
- Advanced Admin Panel: Manage your store efficiently with over 1,000 functionalities at your fingertips.
- Responsive and Customizable Design: Create a unique online storefront that looks great on any device.
- Enhanced Sales and Marketing Tools: Boost your sales with features like abandoned cart recovery.
- International Support: Cater to a global audience with multi-language and multi-currency options.
- Robust Security Measures: Protect customer data with advanced security features.
Summer Cart Pricing Options
Summer Cart offers flexible pricing plans to suit different business needs, ranging from $222 to $829 per year. Each tier provides varying levels of features and support, allowing businesses to choose a plan that aligns with their requirements. For detailed pricing information, visit the official Summer Cart Pricing Page.
User Insights on Summer Cart
While specific user reviews are not accessible here, feedback generally highlights Summer Cart’s extensive features and user-friendly interface. Some users note that there may be a learning curve due to the software’s depth, and pricing can be a consideration for some businesses. For detailed testimonials and reviews, it is advisable to consult dedicated review platforms such as G2.
Video Review of Summer Cart
- In-depth Review of Summer Cart E-commerce Software: An extensive analysis discussing the advantages and potential drawbacks.
Alternatives to Summer Cart
If you’re exploring other options besides Summer Cart, consider these popular e-commerce platforms:
- BigCommerce
- Spocket
- Payhip
- Rap Booster
- Weebly Ecommerce
- WooCommerce
- PrestaShop
- OpenCart
- Wix
- Squarespace
These alternatives offer a variety of features and pricing models that might align with your specific business needs.
Explore seamless integrations with Post Affiliate Pro to enhance your affiliate marketing strategies. Discover solutions for e-commerce, email marketing, payments, and more, with easy integrations for platforms like 1&1 E-Shop, 2Checkout, Abicart, and many others. Optimize your affiliate network with these powerful tools.
Discover Post Affiliate Pro's flexible pricing plans tailored to fit your business needs, with options for Pro, Ultimate, and Network packages. Enjoy a free trial with no credit card required, no setup fees, and the freedom to cancel anytime. Benefit from features like unlimited affiliates, advanced reporting, customizable interfaces, and lifetime support. Save up to 20% with annual billing and take advantage of more than 220 integrations. Perfect for businesses seeking to enhance their affiliate marketing efforts. Visit now to find the ideal plan for you!